Notice
Recent Posts
Recent Comments
Link
일 | 월 | 화 | 수 | 목 | 금 | 토 |
---|---|---|---|---|---|---|
1 | 2 | 3 | 4 | 5 | 6 | 7 |
8 | 9 | 10 | 11 | 12 | 13 | 14 |
15 | 16 | 17 | 18 | 19 | 20 | 21 |
22 | 23 | 24 | 25 | 26 | 27 | 28 |
29 | 30 |
Tags
- Programmers
- RVO
- 2294
- 프로그래머스
- C++
- 줄 세우기
- C
- UnrealEngine5
- 언리얼엔진5
- const
- TObjectPtr
- softeer
- 1563
- 백준
- Unreal Engine5
- IFileDialog
- 팰린드롬 만들기
- winapi
- 오블완
- UnrealEngine4
- NRVO
- algorithm
- GeeksForGeeks
- baekjoon
- RootMotion
- 티스토리챌린지
- UE5
- DirectX11
- directx
- Frustum
Archives
- Today
- Total
Game Develop
[Algorithm]Baekjoon 1484번 :: 다이어트 본문
https://www.acmicpc.net/problem/1484
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
|
#include <iostream>
#include <string>
#include <map>
#include <vector>
#include <algorithm>
#include <math.h>
#include <queue>
#include <functional>
#include <sstream>
#include <memory.h>
#include <deque>
#include <set>
#include <unordered_set>
#include <thread>
#include <atomic>
using namespace std;
int g;
vector<long long> v;
int main()
{
ios::sync_with_stdio(false);
cin.tie(NULL);
cout.tie(NULL);
cin >> g;
for (long long i = 0; i <= g; ++i)
{
v.push_back(i * i);
}
int start = 1;
int end = 2;
vector<long long> answers;
while (start + end <= g)
{
if (v[end] - v[start] > g)
{
++start;
}
else if (v[end] - v[start] < g)
{
++end;
}
else
{
answers.push_back(end);
++start;
}
}
if (answers.size() == 0)
{
cout << -1;
}
else
{
for (int i = 0; i < answers.size(); ++i)
{
printf("%lld\n", answers[i]);
}
}
}
|
cs |
투포인터의 반복횟수는 최대 몇인가? 에 대해 고민했던 문제.
챗지피티덕분에 이해할 수 있었다.
코드에서 알 수 있다시피, s + e <= g이다.
즉, 현재무게 + 이전무게는 반드시 g이하이다.
이해 한방에 되는 예시는 아래와 같다.
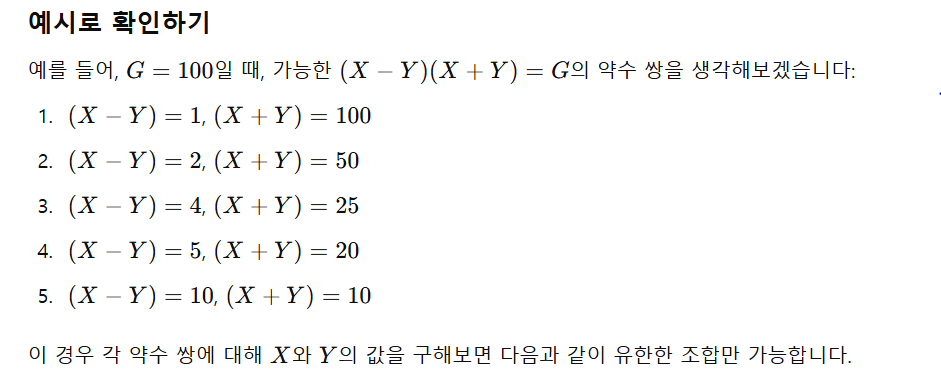
현재무게 ^ 2 - 이전무게 ^ 2 = G 이다.
즉, (현재무게 + 이전무게) * (현재무게 - 이전무게) = G로 다시 고칠 수 있다.
근데 현재무게든, 이전무게든 두 수는 1이상의 자연수이고, 반드시 현재무게가 이전무게보다 더 크다. (G값은 1이상이기 때문에 같을 수 없다)
그렇다면 '현재무게 - 이전무게'는 1보다 더 낮아질 수 없다.
그러니 결국 최대횟수를 결정하는것은 '현재무게 + 이전무게' 이다. 두 수를 곱했을 때, 한쪽 수가 1이니 나머지 수의 최대값은 결국 G값이랑 동일할 수 밖에 없다.
여기서 나머지 수란 X+Y이니, 최대횟수는 X+Y <= G가 되는것이다.
'Algorithm > Baekjoon' 카테고리의 다른 글
[Algorithm] Baekjoon 10597번 : 순열장난 (0) | 2025.03.17 |
---|---|
[Algorithm]Baekjoon 5052번 :: 전화번호 목록 (1) | 2024.11.11 |
[Algorithm]Baekjoon 12978번 :: 스크루지 민호 2 (0) | 2024.11.07 |
[Algorithm]Baekjoon 9207번 :: 페그 솔리테어 (0) | 2024.11.06 |
[Algorithm]Baekjoon 9944번 :: NxM 보드 완주하기 (0) | 2024.11.04 |